Capacitor Native Audio Plugin
Capacitor plugin for native audio engine.
Capacitor V5 - β
Support!
Click on video to see example π₯
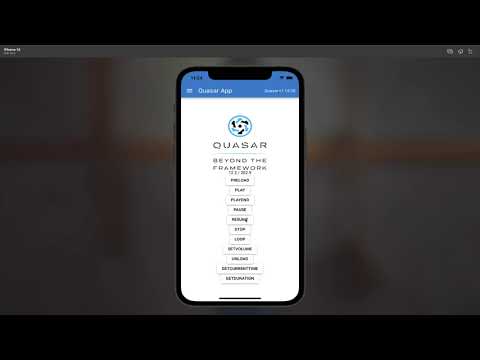
Maintainers
Mainteinance Status: Actively Maintained
Preparation
All audio files must be with the rest of your source files.
First make your sound file end up in your builded code folder, example in folder BUILDFOLDER/assets/sounds/FILENAME.mp3
Then use it in preload like that assets/sounds/FILENAME.mp3
Installation
To use npm
npm install @capgo/native-audio
To use yarn
yarn add @capgo/native-audio
Sync native files
npx cap sync
On iOS, Android and Web, no further steps are needed.
Configuration
No configuration required for this plugin.
Supported methods
Name | Android | iOS | Web |
---|
configure | β
| β
| β |
preload | β
| β
| β
|
play | β
| β
| β
|
pause | β
| β
| β
|
resume | β
| β
| β
|
loop | β
| β
| β
|
stop | β
| β
| β
|
unload | β
| β
| β
|
setVolume | β
| β
| β
|
getDuration | β
| β
| β
|
getCurrentTime | β
| β
| β
|
isPlaying | β
| β
| β
|
Usage
Example repository
import {NativeAudio} from '@capgo/native-audio'
/**
* This method will load more optimized audio files for background into memory.
* @param assetPath - relative path of the file or absolute url (file://)
* assetId - unique identifier of the file
* audioChannelNum - number of audio channels
* isUrl - pass true if assetPath is a `file://` url
* @returns void
*/
NativeAudio.preload({
assetId: "fire",
assetPath: "assets/sounds/fire.mp3",
audioChannelNum: 1,
isUrl: false
});
/**
* This method will play the loaded audio file if present in the memory.
* @param assetId - identifier of the asset
* @param time - (optional) play with seek. example: 6.0 - start playing track from 6 sec
* @returns void
*/
NativeAudio.play({
assetId: 'fire',
// time: 6.0 - seek time
});
/**
* This method will loop the audio file for playback.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.loop({
assetId: 'fire',
});
/**
* This method will stop the audio file if it's currently playing.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.stop({
assetId: 'fire',
});
/**
* This method will unload the audio file from the memory.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.unload({
assetId: 'fire',
});
/**
* This method will set the new volume for a audio file.
* @param assetId - identifier of the asset
* volume - numerical value of the volume between 0.1 - 1.0
* @returns void
*/
NativeAudio.setVolume({
assetId: 'fire',
volume: 0.4,
});
/**
* this method will getΒ the duration of an audio file.
* only works if channels == 1
*/
NativeAudio.getDuration({
assetId: 'fire'
})
.then(result => {
console.log(result.duration);
})
/**
* this method will get the current time of a playing audio file.
* only works if channels == 1
*/
NativeAudio.getCurrentTime({
assetId: 'fire'
});
.then(result => {
console.log(result.currentTime);
})
/**
* This method will return false if audio is paused or not loaded.
* @param assetId - identifier of the asset
* @returns {isPlaying: boolean}
*/
NativeAudio.isPlaying({
assetId: 'fire'
})
.then(result => {
console.log(result.isPlaying);
})
API
configure(...)
configure(options: ConfigureOptions) => Promise<void>
Param | Type |
---|
options | ConfigureOptions |
preload(...)
preload(options: PreloadOptions) => Promise<void>
Param | Type |
---|
options | PreloadOptions |
play(...)
play(options: { assetId: string; time?: number; delay?: number; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; time?: number; delay?: number; } |
pause(...)
pause(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
resume(...)
resume(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
loop(...)
loop(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
stop(...)
stop(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
unload(...)
unload(options: { assetId: string; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; } |
setVolume(...)
setVolume(options: { assetId: string; volume: number; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; volume: number; } |
setRate(...)
setRate(options: { assetId: string; rate: number; }) => Promise<void>
Param | Type |
---|
options | { assetId: string; rate: number; } |
getCurrentTime(...)
getCurrentTime(options: { assetId: string; }) => Promise<{ currentTime: number; }>
Param | Type |
---|
options | { assetId: string; } |
Returns: Promise<{ currentTime: number; }>
getDuration(...)
getDuration(options: { assetId: string; }) => Promise<{ duration: number; }>
Param | Type |
---|
options | { assetId: string; } |
Returns: Promise<{ duration: number; }>
isPlaying(...)
isPlaying(options: { assetId: string; }) => Promise<{ isPlaying: boolean; }>
Param | Type |
---|
options | { assetId: string; } |
Returns: Promise<{ isPlaying: boolean; }>
addListener('complete', ...)
addListener(eventName: "complete", listenerFunc: CompletedListener) => Promise<PluginListenerHandle> & PluginListenerHandle
Listen for complete event
Param | Type |
---|
eventName | 'complete' |
listenerFunc | CompletedListener |
Returns: Promise<PluginListenerHandle> & PluginListenerHandle
Since: 5.0.0
Interfaces
ConfigureOptions
Prop | Type |
---|
fade | boolean |
focus | boolean |
background | boolean |
PreloadOptions
Prop | Type |
---|
assetPath | string |
assetId | string |
volume | number |
audioChannelNum | number |
isUrl | boolean |
PluginListenerHandle
Prop | Type |
---|
remove | () => Promise<void> |
CompletedEvent
Prop | Type | Description | Since |
---|
assetId | string | Emit when a play completes | 5.0.0 |
Type Aliases
CompletedListener
(state: CompletedEvent): void