MUI-datatable
Library to easily generate complex datatables in MUI
Installation
Using npm:
npm i @leandri/mui-datatable
Example
- Import the required type renderers:
import { text } from '@leandri/mui-datatable'
- Define the data:
const data = [
{ id: 1, firstname: 'Olivia', lastname: 'Thompson' },
{ id: 2, firstname: 'Emma', lastname: 'Jones' },
{ id: 3, firstname: 'Stephen', lastname: 'Roberts' },
]
- Define the columns:
const columns = [
text({ key: 'id', name: 'ID' }),
text({ key: 'firstname', name: 'First Name' }),
text({ key: 'lastname', name: 'Last Name' }),
]
- Add the datatable:
import { Datatable } from '@leandri/mui-datatable'
const Home = () => (
<Paper>
<Datatable rows={data} columns={columns} />
</Paper>
)
Type renderers
Text
Props
Name | Type | Required | Default |
---|
key | number | string | Required | - |
name | string | Required | - |
formatter | function | Optional | - |
align | align | Optional | left |
Example
import { text } from '@leandri/mui-datatable'
const rows = [
{ id: 1, firstname: 'Olivia', lastname: 'Thompson' },
{ id: 2, firstname: 'Emma', lastname: 'Jones' },
{ id: 3, firstname: 'Stephen', lastname: 'Roberts' },
]
const columns = [
text({ key: 'id', name: 'ID' }),
text({ key: 'firstname', name: 'First Name' }),
text({ key: 'lastname', name: 'Last Name' }),
text({
key: 'fullname',
name: 'Full Name',
formatter: (row) => `${row.firstname} ${row.lastname}`,
align: 'justify',
}),
]
Output
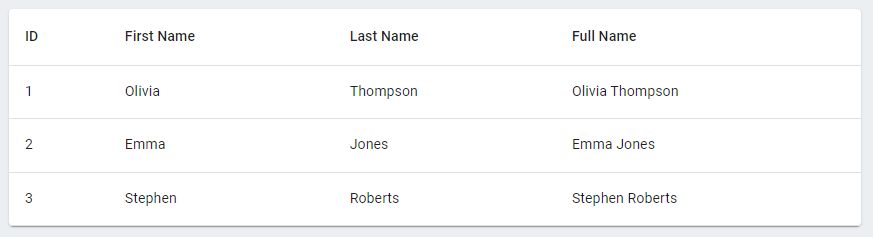
Number
Props
Name | Type | Required | Default |
---|
key | number | string | Required | - |
name | string | Required | - |
align | align | Optional | right |
Example
import { number } from '@leandri/mui-datatable'
const rows = [
{ id: 1, firstname: 'Olivia', age: 18 },
{ id: 2, firstname: 'Emma', age: 30 },
{ id: 3, firstname: 'Stephen', age: 28 },
]
const columns = [
// ...others
number({
key: 'age',
name: 'Age',
align: 'left',
}),
]
Output
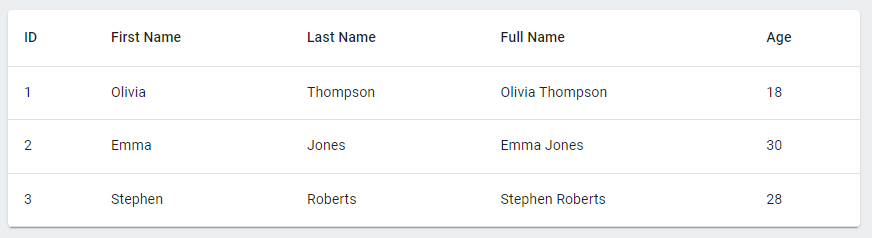
Decimal
Props
Name | Type | Required | Default |
---|
key | number | string | Required | - |
name | string | Required | - |
precision | number | Optional | 2 |
prefix | string | Optional | - |
suffix | string | Optional | - |
align | align | Optional | right |
Example
import { decimal } from '@leandri/mui-datatable'
const rows = [
{ id: 1, name: 'Olivia', progress: 62.25, amount: 90720 },
{ id: 2, name: 'Emma', progress: 100, amount: 156000.5 },
{ id: 3, name: 'Stephen', progress: 86, amount: 134060 },
]
const columns = [
// ...others
decimal({
key: 'progress',
name: 'Progress',
precision: 1,
suffix: '%',
align: 'left',
}),
decimal({
key: 'amount',
name: 'Amount',
prefix: '$',
}),
]
Output
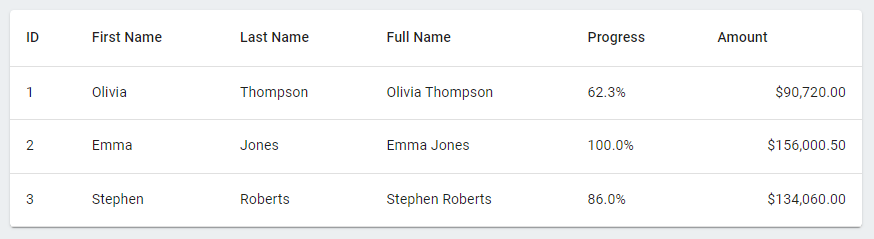
Boolean
Props
Name | Type | Required | Default |
---|
key | number | string | Required | - |
name | string | Required | - |
trueLabel | string | Optional | Yes |
falseLabel | string | Optional | No |
align | align | Optional | right |
Example
import { bool } from '@leandri/mui-datatable'
const rows = [
{ id: 1, firstname: 'Olivia', isAdmin: true },
{ id: 2, firstname: 'Emma', isAdmin: false },
{ id: 3, firstname: 'Stephen', isAdmin: false },
]
const columns = [
// ...others
bool({
key: 'isAdmin',
name: 'Admin',
}),
bool({
key: 'isAdmin',
name: 'Role',
trueLabel: 'Admin',
falseLabel: 'User',
}),
]
Output
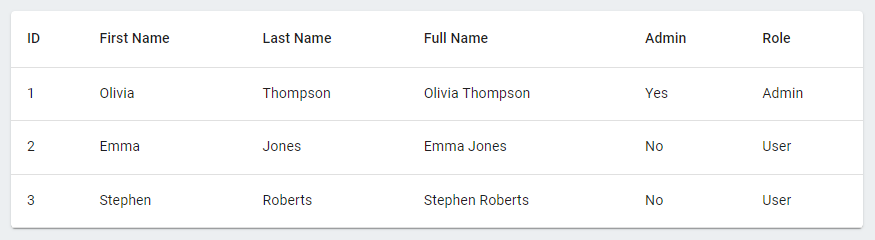