Hola Rx widget is for commercial use and you need a commercial license. Please contact hello@hola.health for more details.
Introduction 🛫
Hola Rx is an electronic prescribing widget and easy to send referrals that can be easily added to any Telehealth, PMS and Web system. Hola Rx is designed and built as per the Electronic Prescribing Conformance stipulated by the Digital Health agency.
Licenses
You need a commercial license to use the Hola Rx widget. Please write to hello@hola.health for more details.
How to use
#1. Installation
Hola Rx is hosted as a package in a private NPM.
npm i @packapilldev/hola-rx
#2. Methods
Method Name | Description |
---|
init | Initializes the widgets and handshakes with the server |
openNewRx | Opens up the new Rx widget |
openEditRx | Opens up the Rx widget for editing script |
openReferral | Opens up the Referral widget |
downloadRx | Downloads the Paper prescription as PDF |
downloadReferral | Downloads the Referral as PDF |
#3. Initialize
You need to initialize the widget using the init method with the provided ClientId, ClientSecret and sandbox (boolean) before invoking the widgets.
//import the package to the appmodule
import { HolaRxModule } from '@packapilldev/hola-rx';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,HolaRxModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
//app.component.ts - import the package in your component page
import { Component, OnInit, ViewChild } from '@angular/core';
import { HolaRx } from '@packapilldev/hola-rx';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
@ViewChild("rxwidget", { static: true }) widget!: HolaRx;
this.widget.init(ClientID, ClientSecret, sandbox).subscribe((res:any)=>{
console.log(res);
});
}
#4. Open Rx Widget to issue a new script
Invoking an Rx widget for a new script for the first time, needs the practice, the practitioner and the patient details.Â
#4.1. openNewRx - Opens up a new Rx widget popup.
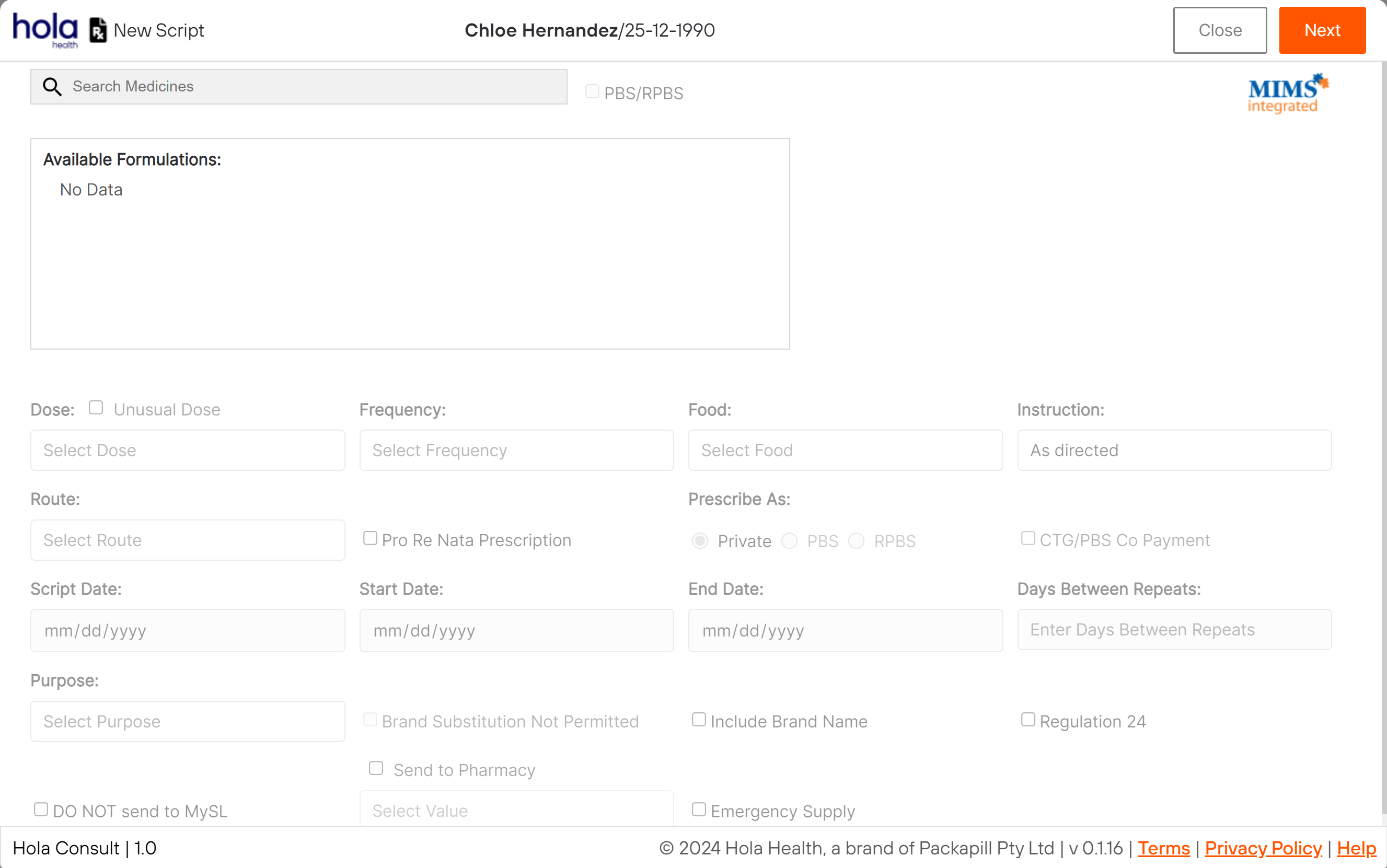
#4.1.1. Input Parameters - Common
Property Name | Friendly Name | Type | Mandatory | Description |
---|
consultationid | Consultation Id | varchar | Yes | Vendor's unique identifier for the consultation |
practiceid | Practice Id | varchar | Conditional | Vendor's unique identifier for the practice/pharmacy |
practitionerid | Practitioner Id | varchar | Conditional | Vendor's unique identifier for the practitioner/pharmacist |
patientid | Patient Id | varchar | Conditional | Vendor's unique identifier for the patient |
practice | Practice | entity | Conditional | Refer Practice Entity |
practitioner | Practitioner | entity | Conditional | Refer Practitioner Entity |
patient | Patient | entity | Conditional | Refer Patient Entity |
rxid | Rx Id | int | Conditional | Hola Rx supplied identifier of the script |
referralid | Referral Id | int | Conditional | Hola Rx supplied identifier of the referrals |
#4.1.2. Input Parameters - Practice Entity
Property Name | Friendly Name | Type | Mandatory | Description |
---|
id | Id | varchar | Yes | Vendor's unique identifier for practice |
name | Name | varchar | Yes | Practice name |
email | Email | varchar | Yes | Practice email address |
phone | Phone | entity | Yes | Practice contact phone number |
timezone | Timezone | varchar | Yes | Timezone of practice. Acceptable values are “Australia/Perth”, “Australia/Eucla”, “Australia/Darwin”, “Australia/Brisbane” , “Australia/Adelaide” , “Australia/Sydney” , “Australia/Lord Howe Island” |
address | Address | entity | Yes | Refer Address Entity |
hpio | HPI-O | int (16 digits) | Conditional | Health Professional Identifier-Organization identifier of the practice. This is mandatory for sending eScripts. |
consent | Consent | bool | Yes | Practice consent to create/update the practice. Accepts “true” or “false” |
#4.1.3. Input Parameters - Practitioner Entity
Property Name | Friendly Name | Type | Mandatory | Description |
---|
id | Id | varchar | Yes | Vendor's unique identifier for practitioner |
firstname | First Name | varchar | Yes | Practitioner's first name |
lastname | Last Name | varchar | Yes | Practitioner's last name |
gender | Gender | varchar | Yes | Birth gender of the practitioner. Accepts “M”, “F” and “NA”. |
dob | Date of Birth | date (yyyy-mm-dd) | Yes | Date of the birth of the practitioner |
email | Email | varchar | Yes | Practitioner email address |
mobile_phone | Mobile Number | varchar | Yes | Practitioner contact mobile number. Format is 0412345678 |
timezone | Timezone | varchar | Yes | Timezone of practice. Acceptable values are “Australia/Perth”, “Australia/Eucla”, “Australia/Darwin”, “Australia/Brisbane” , “Australia/Adelaide” , “Australia/Sydney” , “Australia/Lord Howe Island” |
address | Address | entity | Yes | Refer Address Entity |
registrationnumber | Registration Number | varchar | Yes | AHPRA registration of the practitioner |
prescribernumber | Prescriber Number | int | Yes | Prescriber number of the practitioner |
providernumber | Provider Number | varchar | Yes | Provider number of the practitioner |
hpii | HPI-I | int (16 digits) | Conditional | Health Professional Identifier-Individual. This is mandatory for sending eScripts. |
signature_image | Signature Image | base64 | Conditional | Digital Signature of the practitioner. This is mandatory for sending pathology referrals. This can be added to the paper script as well. |
entity_id | eRx Entity ID | varchar | Yes | Physician eRx entity ID |
consent | Practice Consent | bool | Yes | Practitioner consent to create/update the practitioner. Accepts “true” or “false” |
#4.1.4. Input Parameters - Patient Entity
Property Name | Friendly Name | Type | Mandatory | Description |
---|
id | Id | varchar | Yes | Vendor's unique identifier of patient |
firstname | First Name | varchar | Yes | Patient's first name |
lastname | Last Name | varchar | Yes | Patient's last name |
gender | Gender | varchar | Yes | Birth gender of the patient. Accepts “M”, “F” and “NA”. |
dob | Date of Birth | date (yyyy-mm-dd) | Yes | Date of the birth of the patient |
email | Email | varchar | Yes | Patient email address |
mobile_phone | Mobile Number | varchar | Yes | Patient contact mobile number. Format is 0412345678 |
timezone | Timezone | varchar | Yes | Timezone of practice. Acceptable values are “Australia/Perth”, “Australia/Eucla”, “Australia/Darwin”, “Australia/Brisbane” , “Australia/Adelaide” , “Australia/Sydney” , “Australia/Lord Howe Island” |
address | Address | entity | Yes | Refer Address Entity. |
medicare | Medicare | entity | Conditional | Either Medicare or IHI or Address is mandatory to send eScripts. Refer Medicare Entity. |
ihi | IHI | int (16 digits) | Conditional | Individual Health Identifier of the patient. Either Medicare or IHI or Address is mandatory to send eScripts. |
escript_consent | EScript Consent | bool | Yes | Patient's consent to send eScripts. Accepts “true” or “false” |
#4.1.5. Input Parameters - Address Entity
Property Name | Friendly Name | Type | Mandatory | Description |
---|
addressline1 | Address Line 1 | varchar | Yes | Address Line 1 |
addressline2 | Address Line 2 | varchar | No | Address Line 2 |
suburb | Suburb | varchar | Yes | Suburb |
postcode | Post Code | int | Yes | Valid Australian post code |
state | State Short Code | varchar | Yes | Australian state short codes. Accepts “SA”, “TAS”, "VIC", "WA", "NT", "ACT", "NSW", "QLD" |
#4.1.6. Input Parameters - Medicare Entity
Property Name | Friendly Name | Type | Mandatory | Description |
---|
number | Number | int | Yes | Medicare number |
reference | Reference | int | Yes | Medicare individual reference number |
expiry | Expiry | date (mm/yyyy) | Yes | Medicare expiry |
#4.1.7. Output Parameters - Rx response
Property Name | Friendly Name | Type | Description |
---|
consultationid | Consultation Id | varchar | Vendor's unique identifier for the consultation |
practiceid | Practice Id | varchar | Vendor's unique identifier for the practice/pharmacy |
practitionerid | Practitioner Id | varchar | Vendor's unique identifier for the practitioner/pharmacist |
patientid | Patient Id | varchar | Vendor's unique identifier of patient |
rx | Rx | entity | Refer Rx entity |
status | Status | varchar | Response status. Return values are “success” or “error” |
error | Error | entity | Refer Error entity (may contain multiple values) |
response | Response | entity | Refer Response entity |
#4.1.8. Output Parameters - Rx Entity
Property Name | Friendly Name | Type | Description |
---|
id | Id | int | Hola Rx supplied identifier of the script |
name | Name | varchar | Drug name |
generic_name | Generic Name | varchar | Drug's generic name |
instructions | Instructions | varchar | Medication Instructions |
quantity | Quantity | int | Drug quantity |
repeats | Repeats | int | Drug repeats if there is any. |
scid | SCID | varchar | eRx script/token identifier |
type | Type | varchar | Type of script. it returns "paper" for paper prescription or “escript” for eScripts |
state | State | varchar | Returns the state of the Rx. Possible values are “draft”, “prescribed”, “ceased” and “cancelled” |
#4.1.9. Output Parameters - Error Entity
Property Name | Friendly Name | Type | Description |
---|
error_code | Error Code | varchar | Identifier of the error |
error_message | Error Message | varchar | High level overview of the error |
#4.1.10. Output Parameters - Response Entity
Property Name | Friendly Name | Type | Description |
---|
response_code | Response Code | varchar | Identifier of the response (reserved for future) |
response_message | Response Message | varchar | High level overview of the response |
Code Snippets
details = {
consultationid: "1",
practiceid: "",
practitionerid: "",
patientid: "",
referralid: "",
rxid: "",
practice: {
id: "225",
name: "MedAdvisor1",
email: "noreply@hola.health",
phone: "0412345678",
timezone: "Australia/Perth",
address: {
addressline1: "79 St Georges Terrace",
addressline2: "",
suburb: "Perth",
postcode: "6000",
state: "WA"
},
hpio: "8003627500000328",
consent: true
},
practitioner: {
id: "225",
firstname: "Jane",
lastname: "Blogs",
gender: "M",
dob: "1970-01-01",
email: "noreply@hola.health",
mobile_phone: "0412345678",
timezone: "Australia/Perth",
address: {
addressline1: "79 St Georges Terrace",
addressline2: "",
suburb: "Perth",
postcode: "6000",
state: "WA"
},
registrationnumber: "",
prescribernumber: "1278451",
providernumber: "",
hpii: "8003610833334093",
signature_image: "",
entity_id:"AB123",
consent: true
},
patient: {
id: "225",
firstname: "James",
lastname: "Jayaraj",
gender: "M",
dob: "1970-01-01",
email: "noreply@hola.health",
mobile_phone: "0412345678",
timezone: "Australia/Perth",
address: {
addressline1: "23 Narrow Lane",
addressline2: "",
suburb: "Mannum",
postcode: "5238",
state: "SA"
},
medicare: {
number: "2950249921",
reference: "1",
expiry: "01/2022"
},
ihi: "8003608500012216",
escript_consent: true
}
}
this.widget.openNewRx(this.details).subscribe((res:any)=>{
console.log(res);
});
#5 Open Rx Widget to edit a script
Invoking an Rx widget for editing a script only needs the patient ID, practice ID, practitioner ID and rx ID. However, it does accept full parameters as mentioned in #4 above to update any of the details.
#5.1. openEditRx - Opens up a Rx widget popup in Edit mode.
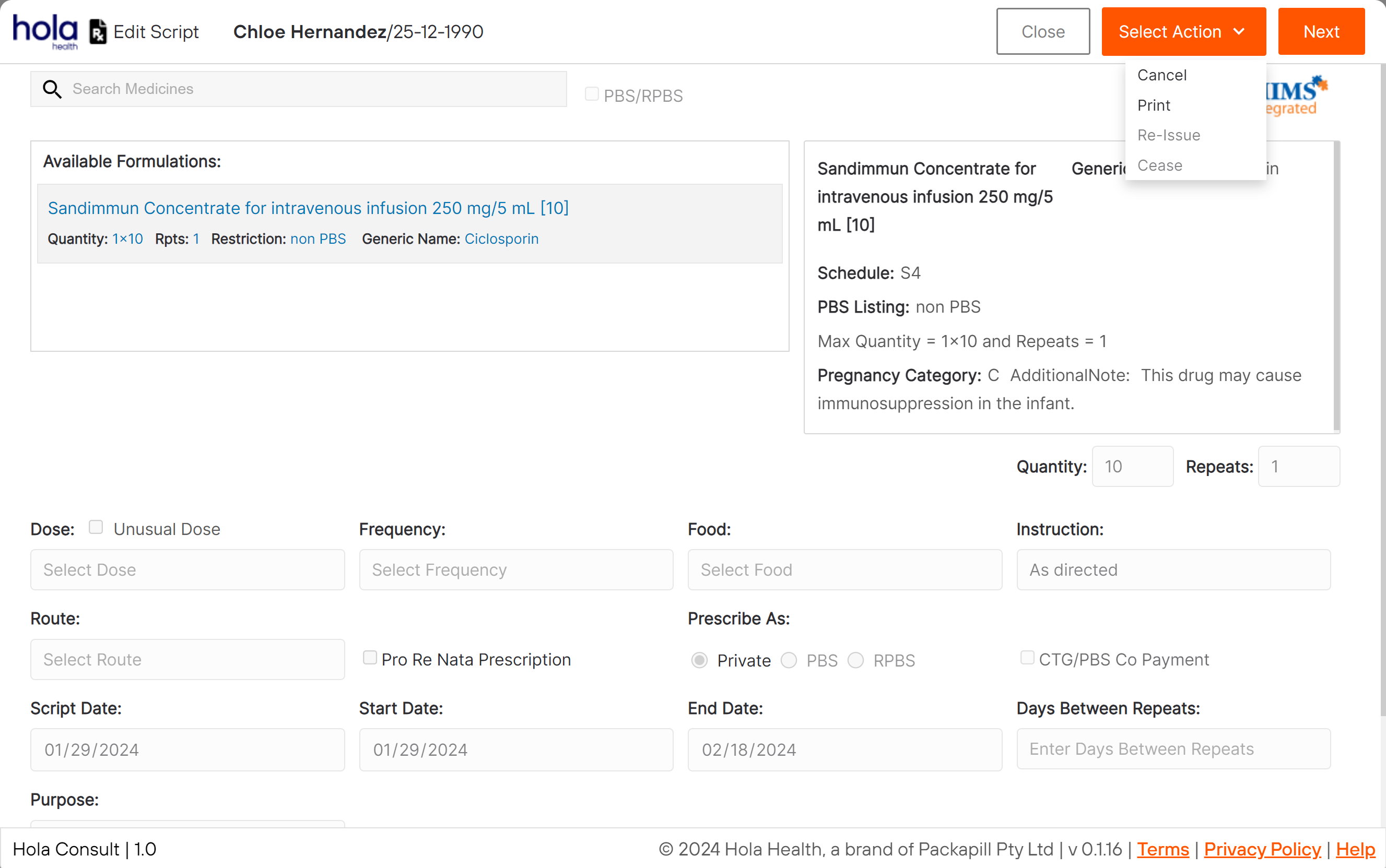
Code Snippets
details = {
consultationid: "1",
practiceid: "",
practitionerid: "",
patientid: "",
referralid: "",
rxid: ""
}
this.widget.openEditRx(this.details).subscribe((res:any)=>{
console.log(res);
});
#6 Open Referral Widget to send pathology referrals
Invoking the Referral widget for sending referrals to the patient for the first time, needs the practice, the practitioner and the patient details. If the details are initialized earlier, you can send only the patient ID, practice ID and practitioner ID.
#6.1. openReferral - Opens up the referral widget popup.
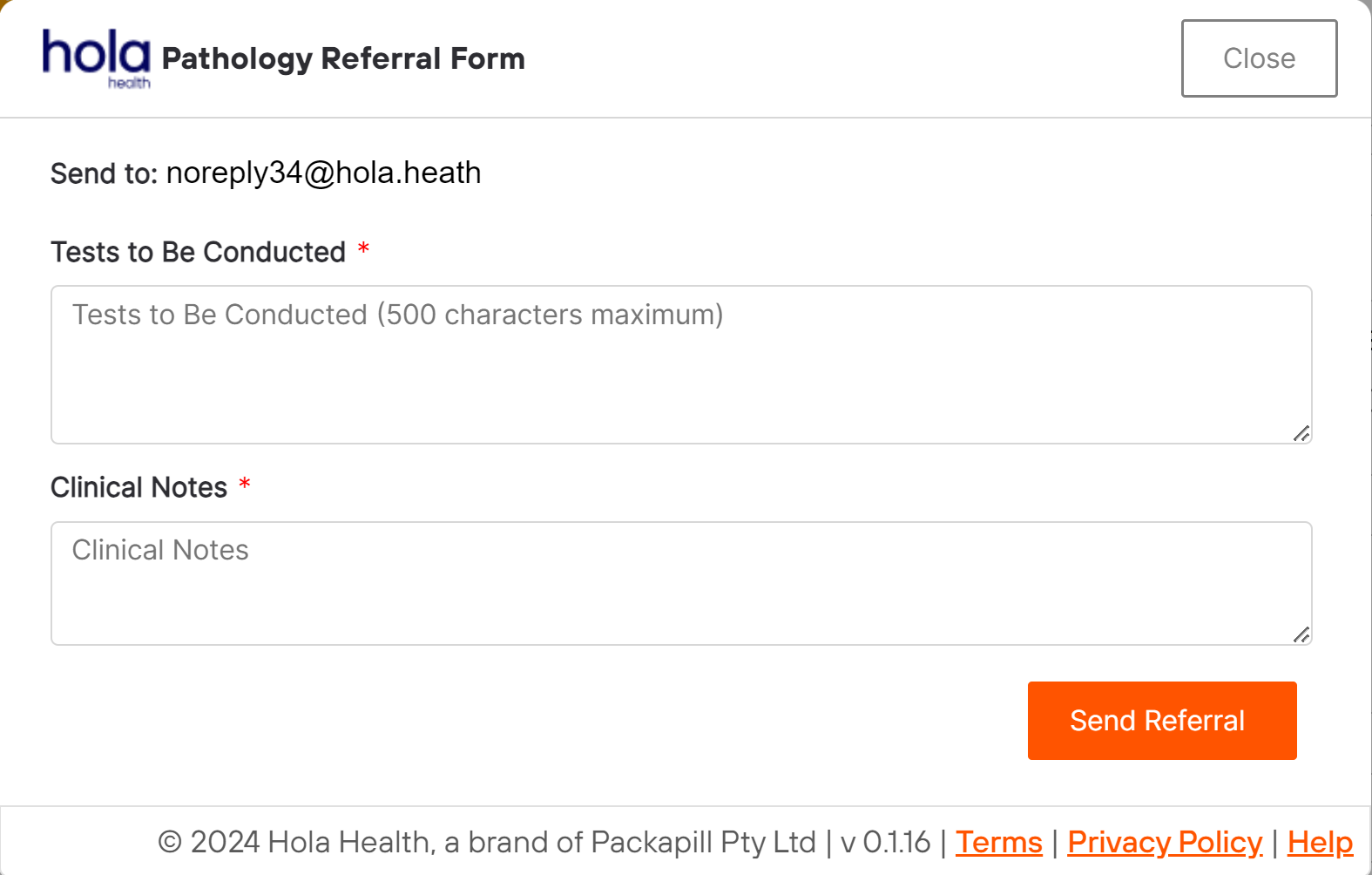
#6.1.1. Output Parameters - Referral response
Property Name | Friendly Name | Type | Description |
---|
consultationid | Consultation Id | varchar | Vendor's unique identifier for the consultation |
practiceid | Practice Id | varchar | Vendor's unique identifier for the practice/pharmacy |
practitionerid | Practitioner Id | varchar | Vendor's unique identifier for the practitioner/pharmacist |
patientid | Patient Id | varchar | Vendor's unique identifier of patient |
referral | Referral | entity | Refer Referral entity |
status | Status | varchar | Response status. Return values are “success” or “error” |
error | Error | entity | Refer Error entity (may contain multiple values) |
response | Response | entity | Refer Response entity |
#6.1.2. Output Parameters - Referral Entity
Property Name | Friendly Name | Type | Description |
---|
id | Id | int | Hola Rx supplied identifier of the referral |
test | Test | varchar | Test to be conducted |
clinical_notes | Clinical Notes | varchar | Clinical notes for the referral request |
#6.1.3. Output Parameters - Error Entity
Property Name | Friendly Name | Type | Description |
---|
error_code | Error Code | varchar | Identifier of the error |
error_message | Error Message | varchar | High level overview of the error |
#6.1.4. Output Parameters - Response Entity
Property Name | Friendly Name | Type | Description |
---|
response_code | Response Code | varchar | Identifier of the response (reserved for future) |
response_message | Response Message | varchar | High level overview of the error |
Code Snippets
details = {
consultationid: "1",
practiceid: "",
practitionerid: "",
patientid: ""
}
this.widget.openReferral(this.details).subscribe((res:any)=>{
console.log(res);
});
#7 Downloads
#7.1. downloadRx - Downloads the paper prescription by rxid in PDF format. It can be used to link the prescription from the front-end.
#7.1.1. Output Parameters - Download Rx response
Property Name | Friendly Name | Type | Description |
---|
status | Status | varchar | Response status. Return values are “success” or “error” |
error | Error | entity | Refer Error entity (may contain multiple values) |
response | Response | entity | Refer Response entity |
#7.1.2. Output Parameters - Error Entity
Property Name | Friendly Name | Type | Description |
---|
error_code | Error Code | varchar | Identifier of the error |
error_message | Error Message | varchar | High level overview of the error |
#7.1.3. Output Parameters - Response Entity
Property Name | Friendly Name | Type | Description |
---|
response_code | Response Code | varchar | Identifier of the response (reserved for future) |
response_message | Response Message | varchar | High level overview of the response |
Code Snippets
this.widget.downloadRx(this.rxId).subscribe((res:any)=>{
console.log(res);
});
#7.2. downloadReferral - Downloads the pathology referral form by referralID in PDF format.
Same as #7.1.1, #7.1.2, and #7.1.3 output parameters.
Code Snippets
this.widget.downloadReferral(this.referralId).subscribe((res:any)=>{
console.log(res);
});
© 2024 Hola Health, a brand of Packapill Pty Ltd | v 0.1.16 |