1.0.3 • Published 7 years ago
rx-graceful v1.0.3
rx-graceful 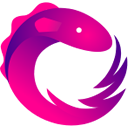
Makes RxJS Subjects graceful. Why? Because...
- Exceptions usually terminate Subjects and often swallow errors.
- It's naive to be confident our code does not contain an exception.
Installation
npm install rx-graceful
Example
'use strict'
const Rx = require('rxjs')
require('rx-graceful')(Rx)
const $ = new Rx.Subject()
$
// (1) catches exceptions inside map
.map(() => { throw Error() })
// (2) catches rejections inside flatMap
.flatMap(x => Promise.reject())
// (3) catches exceptions inside async functions (as these are rejections as well)
.flatMap(async x => { throw Error() })
.subscribe(x => {
// (4) catches exceptions inside the subscription handler
throw Error()
}, e => {
// Handles errors (1), (2), (3), (4)
})
$.next('some value')
Future improvements
- Use lettable operators instead of mutating
Subject.prototype
, as soon as they're out of beta